KK Star Ratings is very familiar to WordPress users; it helps add star ratings to posts and displays beautiful star ratings in Google search results.
It wouldn’t be an issue if the ratings were genuine, but in some cases, competitors might try to sabotage by giving all 1-star ratings. As a result, the display on Google looks bad, significantly affecting search results and user perception when they see a post with only 1-star ratings.
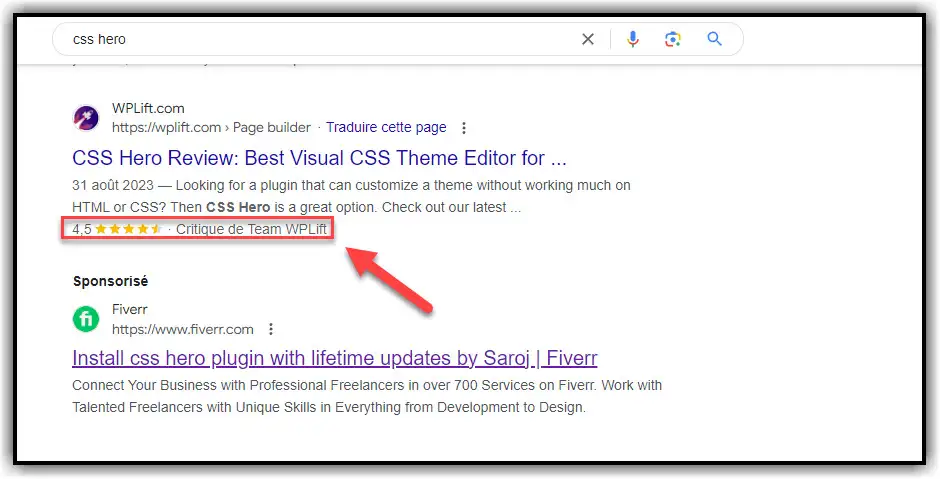
In this article, I will share with you a code snippet that automatically converts ratings below 4 stars to 4–5 stars. The goal is not to make everything 5 stars but to set the ratings between 4 and 5 to make them look more authentic.
Code to Increase Star Ratings to 4>5 Stars
Add the following code to the functions.php
file located in wp-content/themes/[your theme]/functions.php
.
/*
* Set star ratings to 4>5 stars for KK Star Rating
* Author: ohhmua.com
*/
add_action( 'wp_ajax_update_kk_star_rating', 'devvn_update_kk_star_rating_func' );
function devvn_update_kk_star_rating_func() {
global $wpdb;
$posts_with_low_avg = $wpdb->get_results("
SELECT post_id, meta_value as avg_rating
FROM $wpdb->postmeta
WHERE meta_key = '_kksr_avg'
AND CAST(meta_value AS DECIMAL(3,2)) < 4
");
$posts_with_low_avg_table = array();
if (!empty($posts_with_low_avg)) {
foreach ($posts_with_low_avg as $post) {
$post_id = $post->post_id;
$count = (int) get_post_meta($post_id, '_kksr_count_default', true);
$old_avg = (float) get_post_meta($post_id, '_kksr_avg_default', true);
$random_rating = mt_rand(40, 50) / 10;
$new_ratings = $count * $random_rating;
$new_avg = $new_ratings / $count;
update_post_meta($post_id, '_kksr_ratings_default', $new_ratings);
update_post_meta($post_id, '_kksr_ratings', $new_ratings);
update_post_meta($post_id, '_kksr_count_default', $count);
update_post_meta($post_id, '_kksr_casts', $count);
update_post_meta($post_id, '_kksr_avg_default', $new_avg);
update_post_meta($post_id, '_kksr_avg', $new_avg);
$posts_with_low_avg_table[] = compact('post_id', 'old_avg', 'new_avg');
}
$mess = '<span style="color: red;">Rating has been updated.</span>';
} else {
$mess = '<span style="color: green;">No posts need updating.</span>';
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<title>Manually Set Ratings for KK Star Rating</title>
<style>
* {
margin: 0;
padding: 0;
border: 0;
font-size: 100%;
font: inherit;
vertical-align: baseline;
line-height: 1;
box-sizing: border-box;
-moz-box-sizing: border-box;
-webkit-box-sizing: border-box;
}
body {
font-size: 14px;
}
.container{
padding: 20px;
max-width: 900px;
width: 100%;
margin: 0 auto;
}
table {
width: 100%;
border: 1px solid #ddd;
border-collapse: collapse;
border-spacing: 0;
}
table td, table th {
padding: 5px;
text-align: center;
border: 1px solid #ddd;
}
h1 {
font-size: 20px;
text-align: center;
margin-bottom: 20px;
}
</style>
</head>
<body>
<div class="container">
<h1><?php echo $mess;?></h1>
<?php
if($posts_with_low_avg_table){
?>
<table>
<thead>
<tr>
<td>ID</td>
<td>Post Title</td>
<td>Old Rating</td>
<td>New Rating</td>
<td>Link to Post</td>
</tr>
</thead>
<tbody>
<?php
foreach ($posts_with_low_avg_table as $item){
extract($item);
?>
<tr>
<td><?php echo $post_id;?></td>
<td><?php echo get_the_title($post_id);?></td>
<td><?php echo $old_avg;?></td>
<td><?php echo $new_avg;?></td>
<td><a href="<?php echo get_the_permalink($post_id);?>" title="View Post" target="_blank">View Post</a></td>
</tr>
<?php
}
?>
</tbody>
</table>
<?php
}
?>
</div>
</body>
</html>
<?php
die();
}
After that, open your browser and access the following URL while logged into your website. This means you must be logged in as an admin for this URL to work.
https://[your-domain]/wp-admin/admin-ajax.php?action=update_kk_star_rating
For example, if my site is ohhmua.com, I would go to:https://ohhmua.com/wp-admin/admin-ajax.php?action=update_kk_star_rating
CSS to Block Low Star Ratings
Additionally, if you have new posts where you don’t want users to give 1, 2, or 3-star ratings but only allow 4 or 5 stars, you can add the following CSS to your website. If you’re not familiar with coding, you can add it by going to the menu: Appearance > Customize > Additional CSS.
.kk-star-ratings .kksr-stars > div > div:nth-child(1),
.kk-star-ratings .kksr-stars > div > div:nth-child(2),
.kk-star-ratings .kksr-stars > div > div:nth-child(3) {
pointer-events: none;
}
Code to Increase Star Ratings and Add Ratings for Posts Without Ratings
This code is more advanced. The code above only helps increase existing ratings. However, the code below will both increase and add ratings for posts that do not have star ratings.
If you’re using this code, remove the previous one and replace it with this code.
/*
* Set star ratings to 4>5 stars for kk star rating
* This code also adds ratings to posts that haven't been rated yet
* Author: ohhmua.com
*/
add_action( 'wp_ajax_update_kk_star_rating', 'devvn_update_kk_star_rating_func' );
function devvn_update_kk_star_rating_func() {
global $wpdb;
$posts_with_low_avg = $wpdb->get_results("
SELECT p.ID as post_id, pm.meta_value as avg_rating
FROM $wpdb->posts p
LEFT JOIN $wpdb->postmeta pm
ON p.ID = pm.post_id
AND pm.meta_key = '_kksr_avg'
WHERE (pm.meta_value IS NULL OR CAST(pm.meta_value AS DECIMAL(3,2)) < 4)
AND p.post_type IN ('post')
AND p.post_status = 'publish'
");
$posts_with_low_avg_table = array();
if (!empty($posts_with_low_avg)) {
foreach ($posts_with_low_avg as $post) {
$post_id = $post->post_id;
$count = (int) get_post_meta($post_id, '_kksr_count_default', true);
$old_avg = (float) get_post_meta($post_id, '_kksr_avg_default', true);
if(!$count) $count = rand(10,50); //This part randomizes the total number of ratings if there aren't any. You can change this to a higher number.
$random_rating = mt_rand(40, 50) / 10; //This part defines the desired star rating. For example, to set between 4.5 and 5 stars, change to mt_rand(45, 50) / 10
$new_ratings = $count * $random_rating;
$new_avg = $new_ratings / $count;
update_post_meta($post_id, '_kksr_ratings_default', $new_ratings);
update_post_meta($post_id, '_kksr_ratings', $new_ratings);
update_post_meta($post_id, '_kksr_count_default', $count);
update_post_meta($post_id, '_kksr_casts', $count);
update_post_meta($post_id, '_kksr_avg_default', $new_avg);
update_post_meta($post_id, '_kksr_avg', $new_avg);
$posts_with_low_avg_table[] = compact('post_id', 'old_avg', 'new_avg');
}
$mess = '<span style="color: red;">Ratings updated.</span>';
} else {
$mess = '<span style="color: green;">No posts need updating.</span>';
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<title>Manual Star Rating Update for KK Star Rating</title>
<style>
* {
margin: 0;
padding: 0;
border: 0;
font-size: 100%;
font: inherit;
vertical-align: baseline;
line-height: 1;
box-sizing: border-box;
-moz-box-sizing: border-box;
-webkit-box-sizing: border-box;
}
body {
font-size: 14px;
}
.container{
padding: 20px;
max-width: 900px;
width: 100%;
margin: 0 auto;
}
table {
width: 100%;
border: 1px solid #ddd;
border-collapse: collapse;
border-spacing: 0;
}
table td, table th {
padding: 5px;
text-align: center;
border: 1px solid #ddd;
}
h1 {
font-size: 20px;
text-align: center;
margin-bottom: 20px;
}
</style>
</head>
<body>
<div class="container">
<h1><?php echo $mess;?></h1>
<?php
if($posts_with_low_avg_table){
?>
<table>
<thead>
<tr>
<td>ID</td>
<td>Post Title</td>
<td>Old Rating</td>
<td>New Rating</td>
<td>Link to Post</td>
</tr>
</thead>
<tbody>
<?php
foreach ($posts_with_low_avg_table as $item){
extract($item);
?>
<tr>
<td><?php echo $post_id;?></td>
<td><?php echo get_the_title($post_id);?></td>
<td><?php echo $old_avg;?></td>
<td><?php echo $new_avg;?></td>
<td><a href="<?php echo get_the_permalink($post_id);?>" title="View Post" target="_blank">View Post</a> </td>
</tr>
<?php
}
?>
</tbody>
</table>
<?php
}
?>
</div>
</body>
</html>
<?php
die();
}
In the code above, the line AND p.post_type IN (‘post’)
applies only to posts. If you want to include other post types, such as ‘page’, change that line to AND p.post_type IN (‘post’, ‘page’)
.
That’s it!
Note:
- You can delete the code after it has run.
- Alternatively, you can leave it and run it occasionally.
- If there are too many posts to update, the process may be slow. Please wait until it finishes, then refresh the page until you see the message indicating there are no more posts to update.